Meadow.Foundation.ICs.EEPROM.At24Cxx
At24Cxx | |
---|---|
Status | |
Source code | GitHub |
Datasheet(s) | GitHub |
NuGet package |
The AT24Cxx series of chips provide a mechanism for storing data that will survive a power outage or battery failure. These EEPROMs are available in varying sizes and are accessible using the I2C interface.
Code Example
At24Cxx eeprom;
public override Task Initialize()
{
Resolver.Log.Info("Initialize...");
//256kbit = 256*1024 bits = 262144 bits = 262144 / 8 bytes = 32768 bytes
//if you're using the ZS-042 board, it has an AT24C32 and uses the default value of 8192
eeprom = new At24Cxx(i2cBus: Device.CreateI2cBus(), memorySize: 32768);
return base.Initialize();
}
public override Task Run()
{
Resolver.Log.Info("Write to eeprom");
eeprom.Write(0, new byte[] { 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 });
Resolver.Log.Info("Read from eeprom");
var memory = eeprom.Read(0, 16);
for (ushort index = 0; index < 16; index++)
{
Thread.Sleep(50);
Resolver.Log.Info("Byte: " + index + ", Value: " + memory[index]);
}
eeprom.Write(3, new byte[] { 10 });
eeprom.Write(7, new byte[] { 1, 2, 3, 4 });
memory = eeprom.Read(0, 16);
for (ushort index = 0; index < 16; index++)
{
Thread.Sleep(50);
Resolver.Log.Info("Byte: " + index + ", Value: " + memory[index]);
}
return base.Run();
}
Sample project(s) available on GitHub
Wiring Example
To wire a At24Cxx to your Meadow board, connect the following:
At24Cxx | Meadow Pin |
---|---|
GND | GND |
SCL | D08 (SCL) |
SDA | D07 (SDA) |
VCC | 3V3 |
It should look like the following diagram:
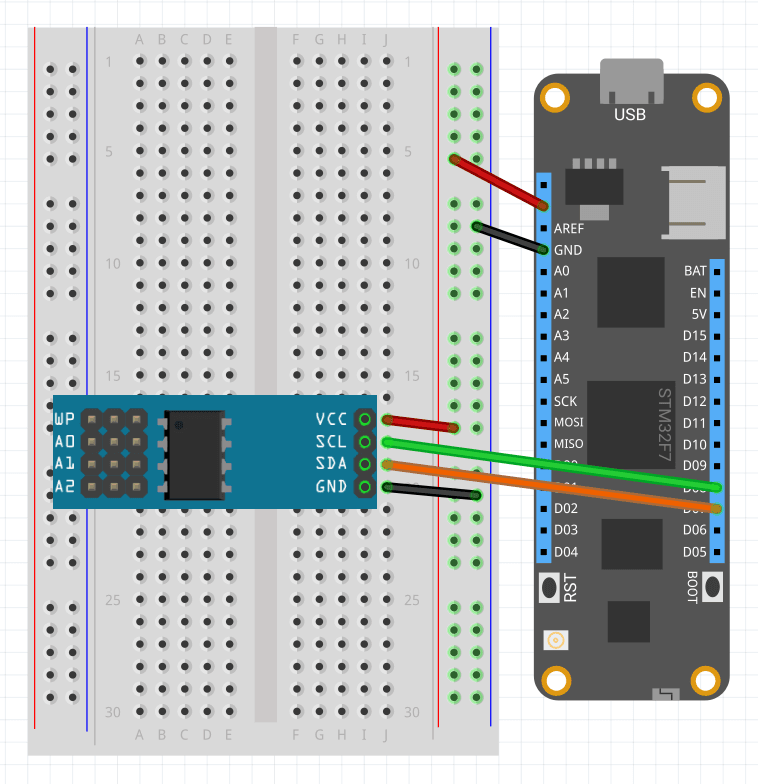