Meadow.Foundation.Motors.Stepper.Uln2003
Uln2003 | |
---|---|
Status | |
Source code | GitHub |
Datasheet(s) | GitHub |
NuGet package |
ULN2003 is a high voltage, high current Darlington array containing seven open collector Darlington pairs. The ULN2003 is often packaged on board used to control stepper motors.
Code Example
Uln2003 stepperController;
public override Task Initialize()
{
stepperController = new Uln2003(
pin1: Device.Pins.D01,
pin2: Device.Pins.D02,
pin3: Device.Pins.D03,
pin4: Device.Pins.D04);
return base.Initialize();
}
public override Task Run()
{
stepperController.Step(1024);
for (int i = 0; i < 100; i++)
{
Resolver.Log.Info($"Step forward {i}");
stepperController.Step(50);
Thread.Sleep(10);
}
for (int i = 0; i < 100; i++)
{
Resolver.Log.Info($"Step backwards {i}");
stepperController.Step(-50);
Thread.Sleep(10);
}
return base.Run();
}
Sample project(s) available on GitHub
Wiring Example
To wire a ULN2003 to your Meadow board, connect the following:
ULN2003 | Meadow Pin |
---|---|
GND | GND |
VCC | 3V3 |
INT1 | D01 |
INT2 | D02 |
INT3 | D03 |
INT4 | D04 |
It should look like the following diagram:
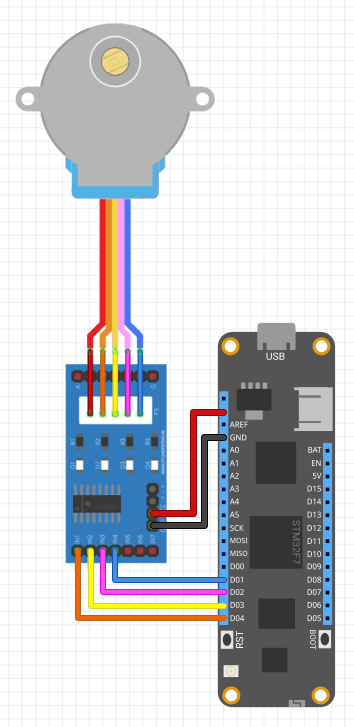
Class Uln2003
This class is for controlling stepper motors that are controlled by a 4 pin controller board.
Assembly: Uln2003.dll
View Source
public class Uln2003 : IDisposable
Implements:
System.IDisposable
Properties
AngularVelocity
Sets the motor speed to revolutions per minute.
View Source
public AngularVelocity AngularVelocity { get; set; }
Mode
Sets the stepper's mode.
View Source
public Uln2003.StepperMode Mode { get; set; }
IsDisposed
Is the object disposed
View Source
public bool IsDisposed { get; }
Methods
Stop()
Stop the motor
View Source
public void Stop()
Step(int)
Moves the motor - If the number is negative, the motor moves in the reverse direction
View Source
public void Step(int steps)
Parameters
Type | Name | Description |
---|---|---|
System.Int32 | steps | Number of steps |
Dispose()
Performs application-defined tasks associated with freeing, releasing, or resetting unmanaged resources.
View Source
public void Dispose()
Dispose(bool)
Dispose of the object
View Source
protected virtual void Dispose(bool disposing)
Parameters
Type | Name | Description |
---|---|---|
System.Boolean | disposing | Is disposing |
Implements
System.IDisposable