Meadow.Foundation.Sensors.Light.Temt6000
Temt6000 | |
---|---|
Status | |
Source code | GitHub |
Datasheet(s) | GitHub |
NuGet package |
The TEMT6000 is an analog ambient light sensor.
Code Example
Temt6000 sensor;
public override Task Initialize()
{
Resolver.Log.Info("Initialize...");
// configure our sensor
sensor = new Temt6000(Device.Pins.A03);
// Example that uses an IObservable subscription to only be notified when the voltage changes by at least 0.5V
var consumer = Temt6000.CreateObserver(
handler: result => Resolver.Log.Info($"Observer filter satisfied: {result.New.Volts:N2}V, old: {result.Old?.Volts:N2}V"),
// only notify if the change is greater than 0.5V
filter: result =>
{
if (result.Old is { } old)
{
return (result.New - old).Abs().Volts > 0.5; // returns true if > 0.5V change.
}
return false;
});
sensor.Subscribe(consumer);
// classical .NET events can also be used:
sensor.Updated += (sender, result) =>
{
Resolver.Log.Info($"Voltage Changed, new: {result.New.Volts:N2}V, old: {result.Old?.Volts:N2}V");
};
return Task.CompletedTask;
}
public override async Task Run()
{
var result = await sensor.Read();
Resolver.Log.Info($"Initial temp: {result.Volts:N2}V");
sensor.StartUpdating(TimeSpan.FromMilliseconds(1000));
}
Sample project(s) available on GitHub
Wiring Example
To wire a Temt6000 to your Meadow board, connect the following:
Temt6000 | Meadow Pin |
---|---|
GND | GND |
SIG | A03 |
VCC | 3V3 |
It should look like the following diagram:
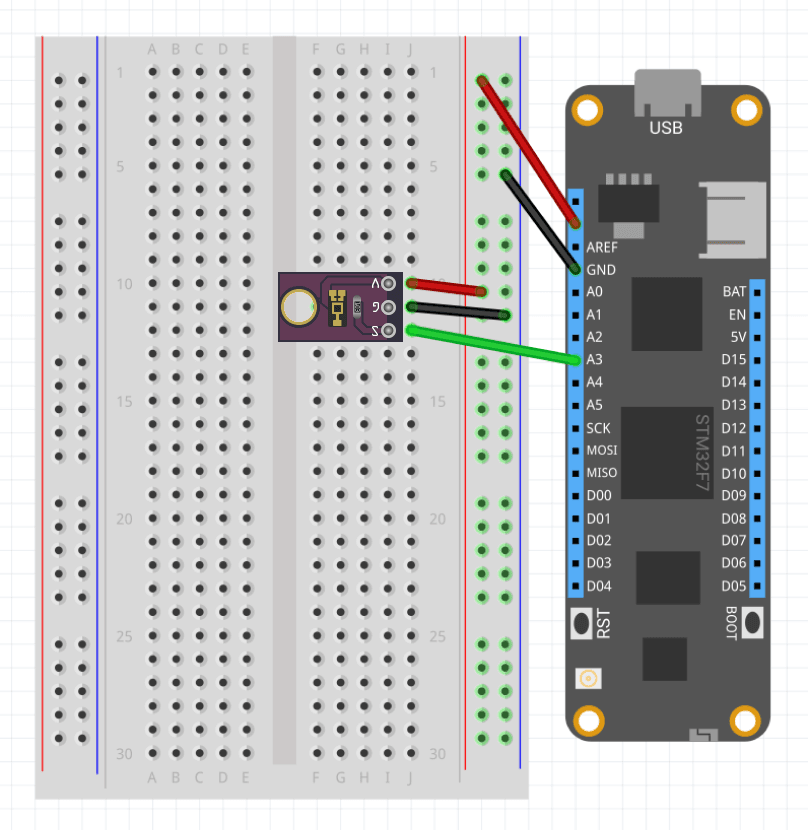
Class Temt6000
Represents a Temt6000 light sensor
Assembly: Temt6000.dll
View Source
Declaration
public class Temt6000 : AnalogSamplingBase, IObservable<IChangeResult<Voltage>>, ISamplingSensor<Voltage>, ISensor<Voltage>, ISensor, ISamplingSensor, IDisposable
Inheritance: System.Object
-> Meadow.Foundation.ObservableBase<UNIT>
Implements:
System.IObservable<Meadow.IChangeResult<Meadow.Units.Voltage>>
, Meadow.Peripherals.Sensors.ISamplingSensor<Meadow.Units.Voltage>
, Meadow.Peripherals.Sensors.ISensor<Meadow.Units.Voltage>
, Meadow.Peripherals.Sensors.ISensor
, Meadow.Peripherals.Sensors.ISamplingSensor
, System.IDisposable
Properties
IsDisposed
Is the object disposed
View Source
Declaration
public bool IsDisposed { get; }
Methods
Dispose()
Performs application-defined tasks associated with freeing, releasing, or resetting unmanaged resources.
View Source
Declaration
public void Dispose()
Dispose(bool)
Dispose of the object
View Source
Declaration
protected virtual void Dispose(bool disposing)
Parameters
Type | Name | Description |
---|---|---|
System.Boolean | disposing | Is disposing |
Implements
System.IObservable<Meadow.IChangeResult<Meadow.Units.Voltage>>
Meadow.Peripherals.Sensors.ISamplingSensor<Meadow.Units.Voltage>
Meadow.Peripherals.Sensors.ISensor<Meadow.Units.Voltage>
Meadow.Peripherals.Sensors.ISensor
Meadow.Peripherals.Sensors.ISamplingSensor
System.IDisposable